A lesser known trick to make use of the HttpContext in asynchronous Event Receivers in SharePoint 2007 or SharePoint 2010 is to define a constructor on the Event Receiver class that assigns the HttpContext to an internal or private member variable. Next you can access it from the method overrides for handling events.
public class MyER1 : SPItemEventReceiver
{
// Local reference to HttpContext during Type Construction so we can use it in the Async Methods
internal HttpContext _ctx = null;
public MyER1()
{
_ctx = HttpContext.Current;
}
public override void ItemAdded(SPItemEventProperties properties)
{
string requestUrl = _ctx.Request.Url.ToString();
}
}
There’s a difference in behavior for Lists and Libraries; the former allows you to also read/write from the Context.Session, while the latter doesn’t allow this (Session is null).
I’ve (ab)used this once on a project where I’d call a custom LayoutPage called with QueryString parameters to force a SPListItem.Update(). In the Event Receiver the code would interact with the values of those parameters.
Side note:
Doubt that there’s official support on this. Feel free to drop a comment with your opinion.
The Office Web Apps allow users without Microsoft Office installed to display or work on Word, Excel or PowerPoint files from the browser. It is a separate installation to your SharePoint Farm and controllable by two Site Collection Features:

When active it will render Office 2007/2010 file formats in the browser, without any requirement to a locally installed Office suite.
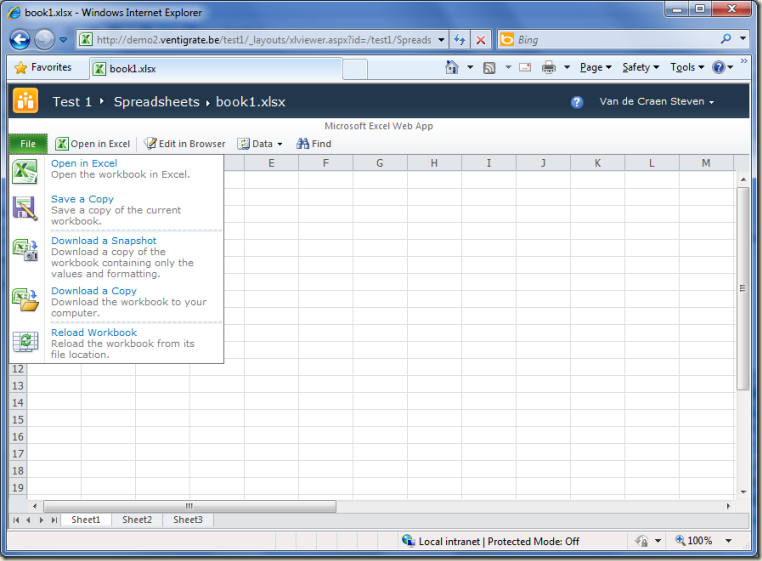
As you notice in the screen there’s no obvious way to create a new document, spreadsheet or presentation. So how would that work ?
The ‘New’ button on libraries has both functionalities; when a local installation of Office is found it will open up the corresponding application, else it will navigate to a page for creating a new file directly from the browser.
Word Web Application:
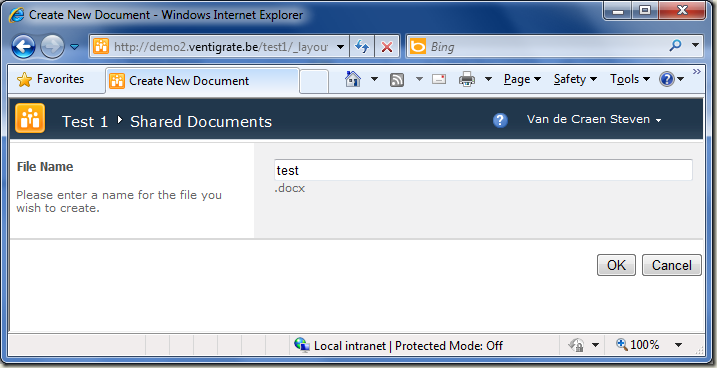
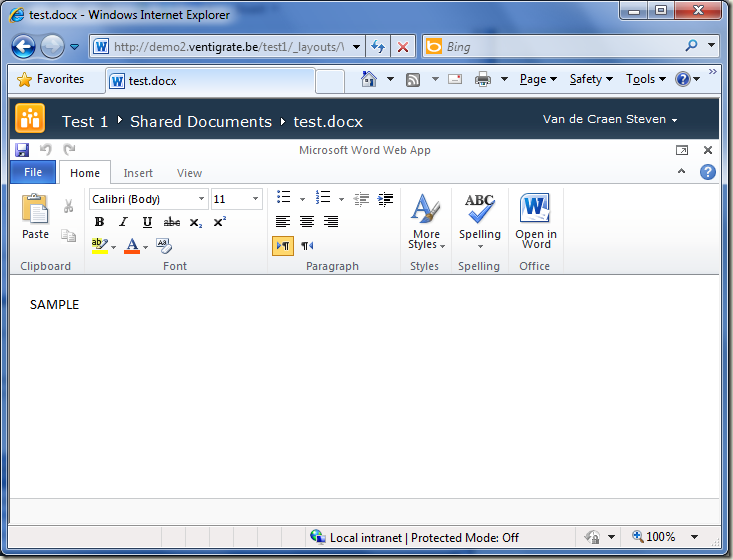
If you’re using a modified document template, the new document will be based on the modified template.
Excel Web Application:
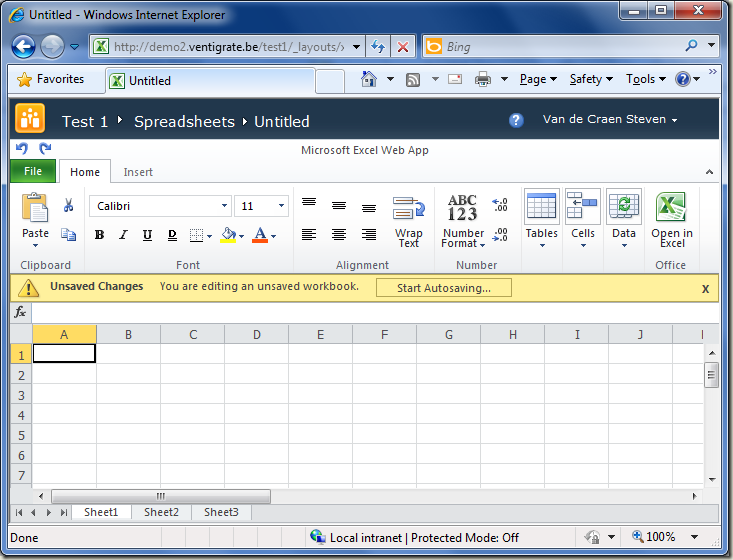
If you’re using a modified spreadsheet template, the new document will NOT be based on that.
PowerPoint Web Application:
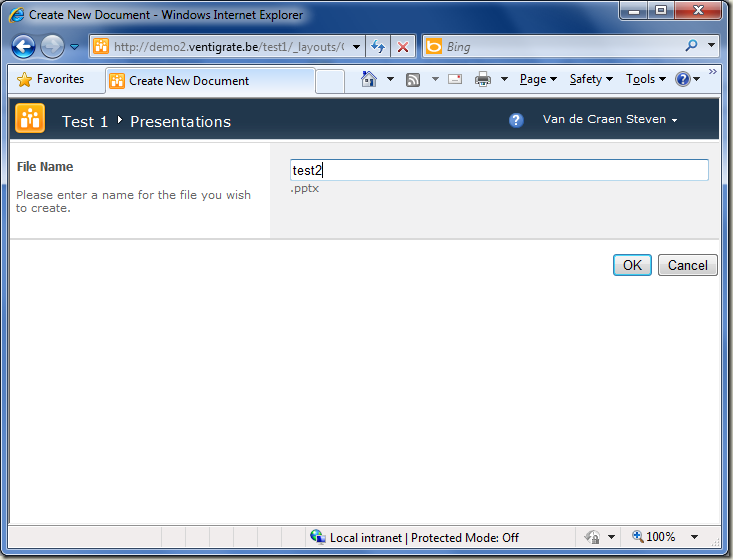
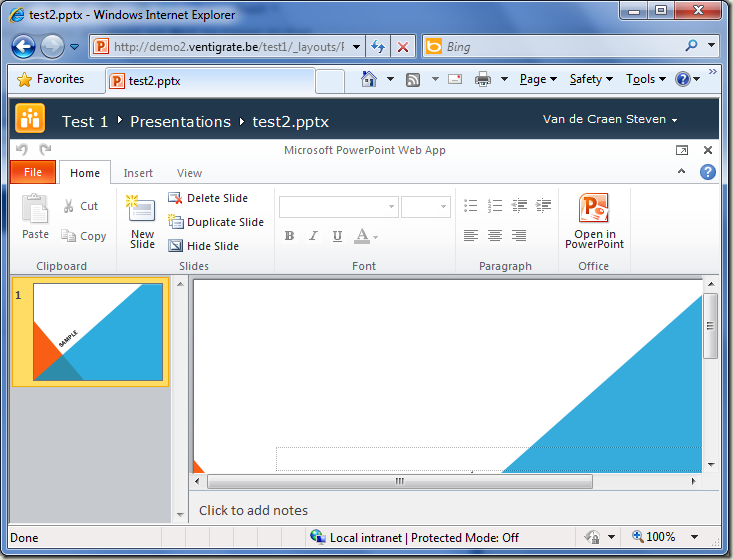
If you’re using a modified presentation template, the new presentation will be based on the modified template.
It’s a shame Excel behaves differently, but other than that it’s a really nice feature !
A feature of using the Remote Blob Storage with SharePoint 2010 (FILESTREAM provider in SQL Server 2008) is that document versions do not always create a new full copy of the original file, as it would in a non-RBS environment.
This is a huge improvement since 5 versions of a 10 MB file where only metadata is changed would result in 50 MB purely for file contents (not counting metadata storage size).
SharePoint 2010 without RBS
The files are stored in the Content Database in [AllDocStreams] in a varbinary field called Content. Each version counts as a record and the Contents field is replicated, even if only a metadata change occurred.
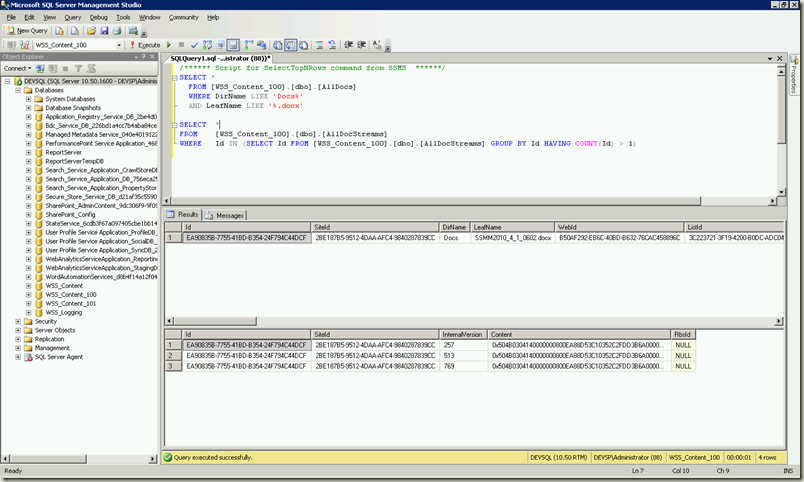
SharePoint 2010 with RBS
The files are stored on the file system. Each file or version record in the Content Database (table [AllDocStreams]) maintains a pointer to the RbsId. As you see in the screen below multiple versions still point to the same identifier of the blob, as long as the actual file hasn’t changed.
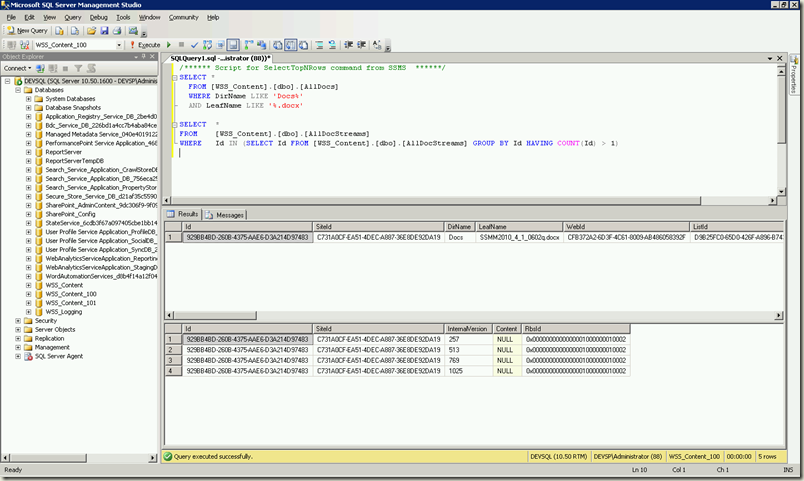
Very nice !