I'm normally not into Office Automation but today I needed to extract all embedded files from a Word Document. Those files were Word, Excel and PDF documents. Luckily the majority were Word documents, because the quick solution I whipped up only works for those types, not for Excel or PDF.
Here's the VBA script that loops through the embedded objects, checks if it's a Word document and goes to save it in a new folder.
Sub ExtractFiles()
'
' ExtractFiles Macro
'
'
Dim shape As InlineShape
Dim folderName As String
Dim a As Document
folderName = Replace(ThisDocument.Name, ".", "_")
MkDir folderName
For Each shape In ThisDocument.InlineShapes
If (shape.Type = wdInlineShapeEmbeddedOLEObject) And (InStr(LCase(shape.OLEFormat.IconLabel), ".doc") > 0) Then
shape.OLEFormat.Object.SaveAs (folderName & "\" & shape.OLEFormat.IconLabel)
End If
Next shape
End Sub
Today I got into testing how to filter a list with a multivalued Choice (or Lookup) column based on a multivalued filter. The setup I'm using is to connect a Choice Filter Web Part with the List View Web Part.
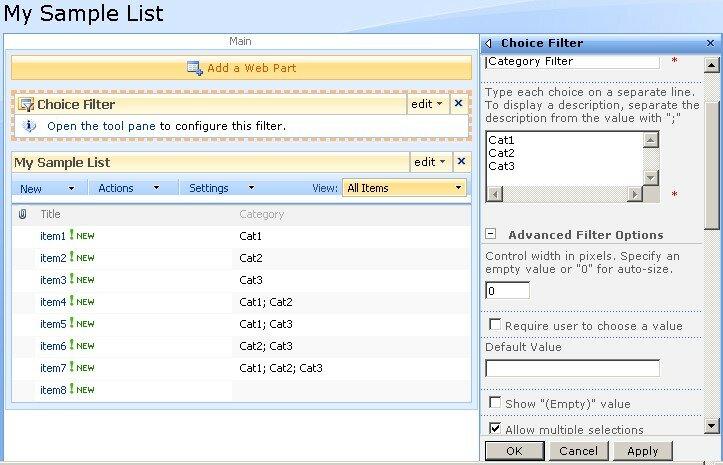
Once you have configured the Filter Web Part and connected it to the List View Web Part the fun starts. Just take a look at the following result rest:
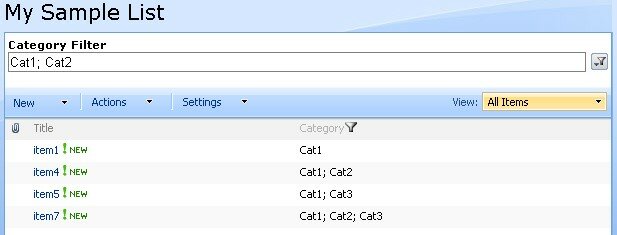
... and compare it with this one:
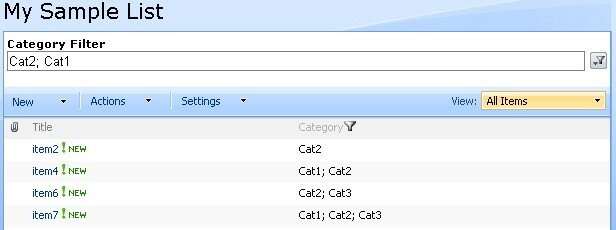
The only difference here is the order of the filter values. But the result set shouldn't be different, should it? What's the logic behind it? Does it merge result sets of individual filter values? Confusing indeed... It does do something 'special' with the first filter value.
note: If you want to change the order of the filter values you can do so by selecting them one at a time: Cat2 > OK > Cat1 > OK
note2: It also works without the 'OK' step :)
Here's an overview (just as a reference really):
FILTER |
RESULT |
Cat1 |
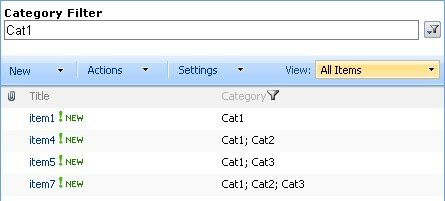 |
Cat2 |
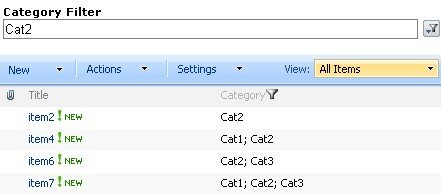 |
Cat3 |
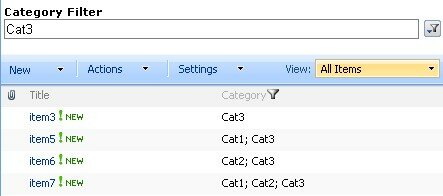 |
Cat1; Cat2 |
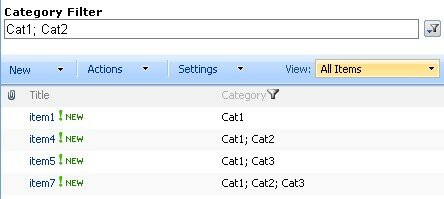 |
Cat2; Cat1 |
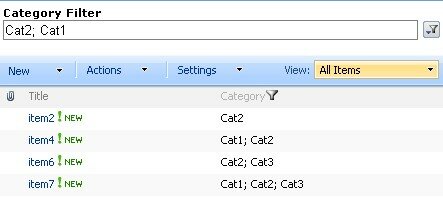 |
Cat1; Cat3 |
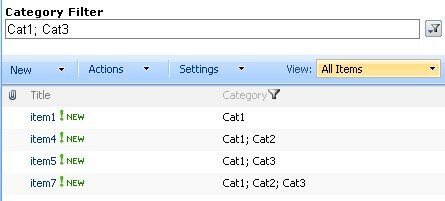 |
Cat3; Cat1 |
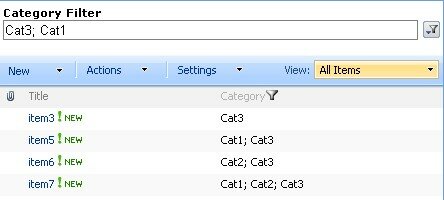 |
Cat2; Cat3 |
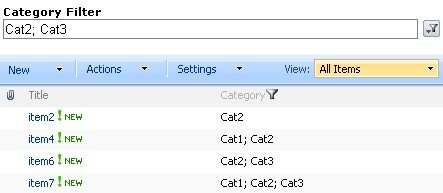 |
Cat3; Cat2 |
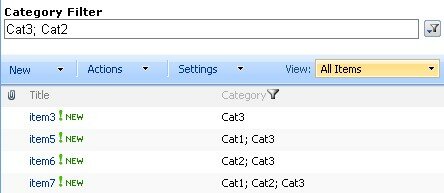 |
Cat1; Cat2; Cat3 |
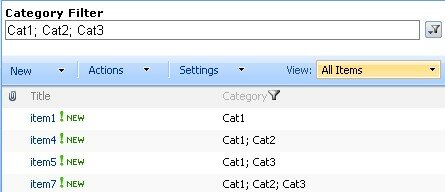 |
Cat1; Cat3; Cat2 |
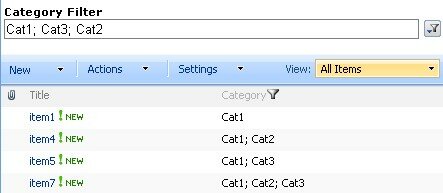 |
Cat2; Cat1; Cat3 |
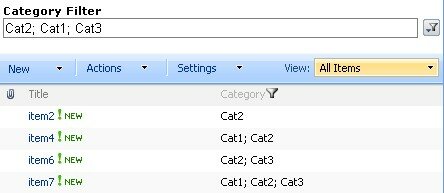 |
Cat2; Cat3; Cat1 |
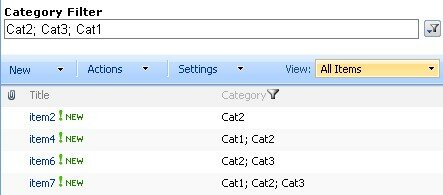 |
Cat3; Cat1; Cat2 |
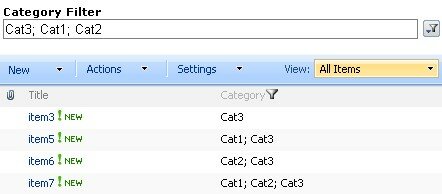 |
Cat3; Cat2; Cat1 |
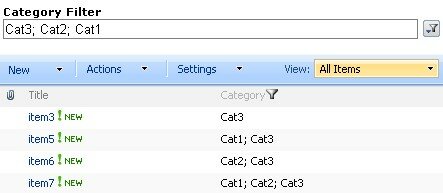 |
(empty) |
(no filtering) |
I think it's safe to say this isn't really a reliable scenario. A better way of filtering can be achieved using the Query String:
http://vmoss/Lists/My%20Sample%20List/AllItems.aspx?View={4960D60A-B5F4-42FF-ABD2-23EB2CFD30E3}&FilterField1=Category&FilterValue1=Cat1&FilterField2=Category&FilterValue2=Cat2&FilterField3=Category&FilterValue3=Cat3
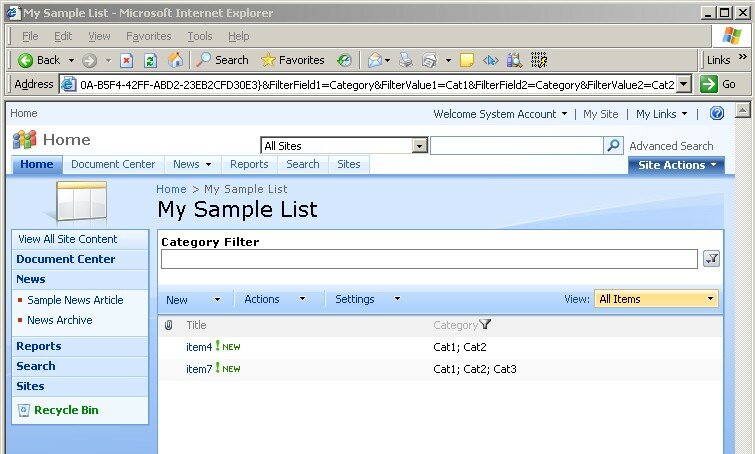
This is the same mechanism as if you would click on a filterable column and specify a value there. Only doesn't it allow you to select multiple values.
This works like an AND filter. There seems to be no way in making it behave like an OR filter, but so be it. It is still way more reliable than usin the Choice Filter Web Part.
note: If you're not doing multivalued filtering both methods are equally reliable !